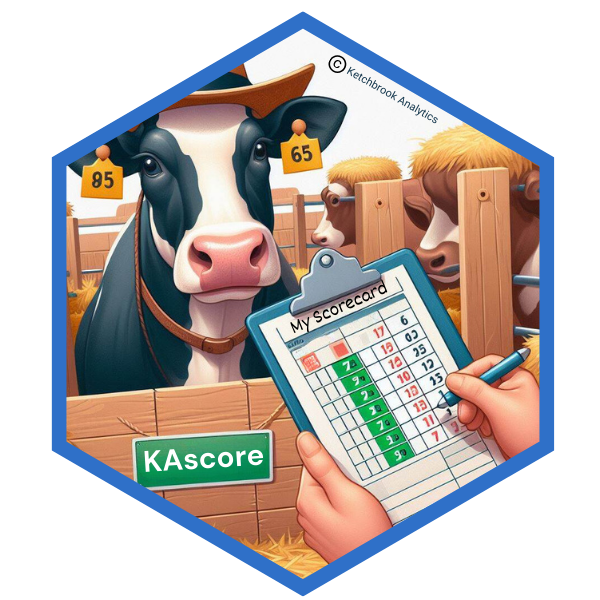
Deployment
deployment.Rmd
The “Build a Scorecard” vignette
demonstrates how to use the scorecard()
function to create
a model object against which predict()
can be called. This
article provides a roadmap for deploying the model as a REST API service
via {plumber}, allowing your
model to be called from any other application that can make API
requests.
Saving the Model Object
The API will need access to the model object generated by the
scorecard()
function. The best way to do this is by saving
the model object to an .RDS file:
saveRDS(scorecard_fit, "scorecard_fit.rds")
Creating the API
The following plumber.R
file sets up an API endpoint
(/predict
) that accepts parameters as inputs to the
scorecard model (in our example, these represent the loan applicant’s
values for industry
and housing_status
), uses
the pre-trained scorecard model to generate predictions and returns
either points or probabilities, depending on how the
type
argument is specified.
# plumber.R
# Make `predict()` method available for object of class "scorecard"
library(KAscore)
# Read model object
scorecard_fit <- readRDS("scorecard_fit.rds")
#* @apiTitle Scorecard Prediction
#* @apiDescription API Service for Scorecard Prediction
#* @apiVersion 1.0
#* Predict points or prob for new loans
#* @param industry (String) The class within the industry independent variable
#* @param housing_status (String) The class within the housing_status independent variable
#* @param type (String) One of "points" or "prob", indicating the type of prediction to return
#* @post /predict
function(industry, housing_status, type) {
new_data <- tibble::tribble(
~industry, ~housing_status,
industry, housing_status
)
predict(
object = scorecard_fit,
newdata = new_data,
type = type
)
}
Testing the API Locally
To launch the API locally, run the following command from the R
console. You can specify the port on the server to launch the API at by
using the port
argument from the
plumber::pr_run()
function. For example:
plumber::pr("plumber.R") |>
plumber::pr_run(port = 8080)
Calling the API
Terminal / Command Prompt
You can use the curl
command to call the API using the
terminal, command prompt, shell, etc.
curl -X POST "http://127.0.0.1:8080/predict?industry=poultry&housing_status=own&type=points
R
As long as the API is running, you can even call it from another R session (using {httr}, for example).
call_api <- function(industry, housing_status, type, port = 8080) {
res <- httr::POST(
glue::glue("http://127.0.0.1:{ port }/predict"),
query = list(
industry = industry,
housing_status = housing_status,
type = type
)
)
res$content |>
rawToChar() |>
jsonlite::fromJSON()
}
# Get points
call_api(
industry = "poultry",
housing_status = "own",
type = "points"
)
## 254
# Get prob
call_api(
industry = "poultry",
housing_status = "own",
type = "prob"
)
## 0.141
Containerizing the API with Docker
In order to safely ship the REST API service we created locally to another machine (e.g., a cloud-based server), we can containerize it using Docker.
Docker is a virtualization software that allows you to package your code, files, and operating system into a fully reproducible environment called a “container”. This ensures that the behavior your software produces on your local computer will be identical to the behavior your software produces on any other server.
The following steps demonstrate how to create a Docker container image that serves our scorecard REST API in a way that can be easily deployed on any server.
Step 1: Create a Dockerfile
A Dockerfile is a set of instructions on how to create the Docker image. For the purposes of deploying your scorecard model, we recommend creating a Dockerfile similar to this one:
# Dockerfile
# Base image
FROM eddelbuettel/r2u:22.04
# Define an argument for the version of KAscore
ARG KASCORE_VERSION=1.2.0
# Set an environment variable for the KAscore path
ENV KASCORE_PATH=KAscore_${KASCORE_VERSION}.tar.gz
# Copy necessary files into the container
COPY scorecard_fit.rds plumber.R ${KASCORE_PATH} .
# Install required R packages
RUN install2.r remotes plumber
# Install KAscore package from tarball provided by Ketchbrook
RUN R -e 'remotes::install_local(Sys.getenv("KASCORE_PATH"))'
# Expose port 3838
EXPOSE 3838
# Define the command to run the R script that launches the API
CMD ["R", "-e", "plumber::pr('plumber.R') |> plumber::pr_run(host = '0.0.0.0', port = 3838)"]
Step 2: Build the Docker image
In your terminal, navigate to the directory containing your
Dockerfile, scorecard_fit.rds
object,
plumber.R
script and KAscore_1.2.0.tar.gz
file
and execute the following command to build the Docker image:
docker build -t scorecard-api .
Step 3: Run the Docker container
After building the Docker image, you can run it using the following command:
docker run -d --rm -p 3838:3838 --name scorecard-api-container scorecard-api
Step 4: Access the API
You can navigate to http://127.0.0.1:3838/__docs__/ in your local web browser to access the interactive API documentation generated by Plumber. This is a convenient way to verify that the API is working as expected.
We can now send requests to http://127.0.0.1:3838/predict:
call_api(
industry = "poultry",
housing_status = "own",
type = "prob",
port = 3838
)
Deployment
There are a multitude of hosting options for deploying a REST API as a service that can be consumed by others. In decades past, organizations may have deployed on their own physical infrastructure. Nowadays most organizations turn to cloud-based services for deploying REST APIs. Such cloud providers include Microsoft Azure, Amazon Web Services, Google Cloud Platform, DigitalOcean, and many more.
Microsoft Azure
Currently, Azure Container Instances is one of the most popular options for deploying API services on the Azure cloud. This tutorial walks you through setting up a containerized REST API service using the Azure portal.
Prior to doing this, however, you will need to push the Docker image you created in Part 2, above, to a container registry. There are multiple container registries where you can push your Docker image to, but perhaps the two most popular for this setup would be Azure Container Registry or Docker Hub.
More Information
For more information on deploying your scorecard as a REST API service on Azure, AWS, GCP, DigitalOcean, or other cloud providers, please reach out to info@ketchbrookanalytics.com. Our team has extensive experience deploying R and Python models as REST API services that can be consumed by others inside and/or outside your organization.