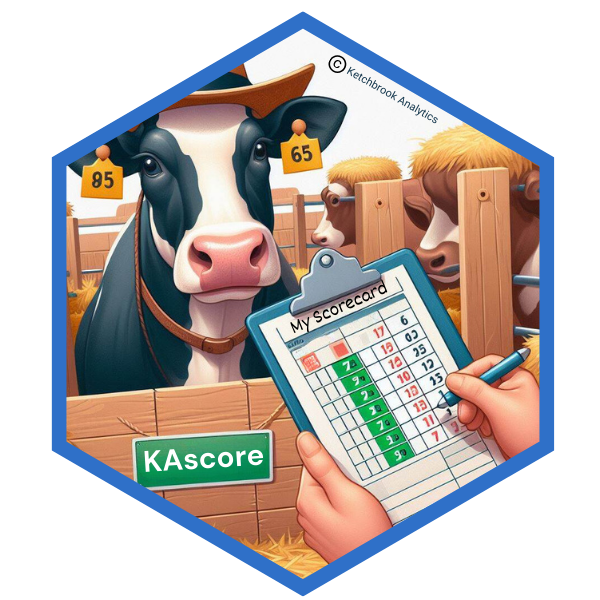
Create a Scorecard Model Object
scorecard.Rd
scorecard()
creates a scorecard object using a fitted logistic model and
card specifications. This function is designed to work with models that have
been created using Weight of Evidence (WoE) features, specifically with a
logistic regression model of class inheriting from "glm"
using the binomial
family and logit link.
Arguments
- fit
A fitted logistic regression model (of class inheriting from
"glm"
) with WoE features. The model should belong to the binomial family and use the logit link.- card
A data frame containing card specifications. The
card
data frame must have the following columns:variable
,class
,woe
, andpoints
. Thevariable
column should be expressed in raw terms, i.e., not as WoE features.
Examples
# Reverse levels of `default_status`
loans$default_status <- factor(loans$default_status, levels = c("good", "bad"))
# Pre-process the data to create WoE features
train <- woe(
data = loans,
outcome = default_status,
predictors = c(industry, housing_status),
method = "replace",
verbose = FALSE
)
# Fit the logistic regression model
my_model <- glm(
formula = default_status ~ .,
data = train,
family = "binomial"
)
# Assume we have the following associated card
my_card <- tibble::tribble(
~variable, ~class, ~woe, ~points,
"industry", "", 1.23, 148,
"industry", "beef", -0.231, 107,
"industry", "dairy", -0.0956, 110,
"industry", "fruit", -0.359, 103,
"industry", "grain", 0.410, 125,
"industry", "greenhouse", -0.511, 99,
"industry", "nuts", -0.288, 105,
"industry", "pork", -0.606, 96,
"industry", "poultry", 0.774, 135,
"industry", "sod", -0.154, 109,
"housing_status", "own", 0.194, 119,
"housing_status", "rent", -0.430, 101
)
# Create the scorecard object
my_scorecard <- scorecard(
fit = my_model,
card = my_card
)