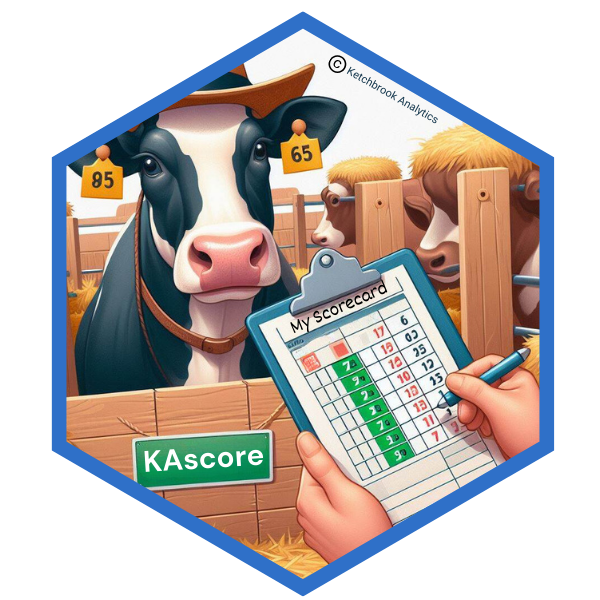
Calculate the Odds by Score
odds.Rd
odds()
calculates the associated odds for each input numeric
score
value provided.
Arguments
- score
(Integer) The scores (number of scorecard points) for which associated odds should be calculated
- tgt_points
(Integer) The target number of points to be used in conjunction with the
tgt_odds
; see Details for more information- tgt_odds
(Numeric) The odds (good:bad) that the
tgt_points
should have; see Details for more information- pxo
(Integer) The default interpretation (via
rate
argument) is the number of points to double the odds. If setting therate
to a value of 3, this would instead be interpreted as the number of points it takes to triple the odds, etc. See Details for more information.- rate
(Numeric) The value to exponentially increase the odds by for the given number of points supplied in the
pxo
argument. Default value is 2 (i.e., double the odds). See Details for more information.
Value
A numeric value (or vector of values) representing the calculated
odds for each input numeric score
value provided.
Details
The tgt_points
and tgt_odds
arguments work together to build a "baseline"
points/odds relationship for your scorecard. For example, a tgt_points
value of 600 and a tgt_odds
value of 30 would be interpreted as "a score
of 600 points would have 30:1 odds of good:bad (i.e., for every 31 loans
that receive a score of 600 points, we expect 30 to be good and 1 to be
bad)."
Once the baseline points & odds are established, the number of points to
exponentially increase the odds and the rate of that exponential increase
(e.g., double, triple, etc.) must be defined. Traditionally, the rate
value is 2, meaning "double" the odds every pxo
number of points.
See below for example values & interpretations:
tgt_points = 300
,tgt_odds = 30
,pxo = 20
,rate = 2
: a score of 300 points has 30:1 odds of good:bad, and the odds double every increase of 20 points (e.g., a score of 320 has 60:1 odds, a score of 340 has 120:1 odds, etc.)tgt_points = 600
,tgt_odds = 25
,pxo = 50
,rate = 3
: a score of 600 points has 25:1 odds of good:bad, and the odds triple every increase of 50 points (e.g., a score of 650 has 75:1 odds, a score of 700 has 225:1 odds, etc.)
References
Siddiqi, Naeem (2017). Intelligent Credit Scoring: Building and Implementing Better Credit Risk Scorecards. 2nd ed., Wiley., pp. 240-241.
Examples
# Use the default odds rate (points to *double* the odds)
# Calculate the associated odds for a score of 375
odds(
score = 375L,
tgt_points = 300L,
tgt_odds = 30,
pxo = 20
)
#> [1] 403.6303
# Specify a different odds rate than the default (points to *triple* the
# odds)
odds(
score = seq.int(from = 500L, to = 650L, by = 5L),
tgt_points = 600L,
tgt_odds = 25,
pxo = 50L,
rate = 3
)
#> [1] 2.777778 3.100342 3.460364 3.862192 4.310682 4.811252 5.369950
#> [8] 5.993526 6.689513 7.466320 8.333333 9.301026 10.381091 11.586576
#> [15] 12.932046 14.433757 16.109850 17.980577 20.068539 22.398961 25.000000
#> [22] 27.903079 31.143273 34.759729 38.796139 43.301270 48.329551 53.941732
#> [29] 60.205617 67.196884 75.000000
# Use it in a {dplyr} chain against a column of scores
df <- tibble::tibble(
scores = c(350, 435, 4800, 515)
)
df |>
dplyr::mutate(
odds = odds(
score = scores,
tgt_points = 400L,
tgt_odds = 20,
pxo = 50,
rate = 3
)
)
#> # A tibble: 4 × 2
#> scores odds
#> <dbl> <dbl>
#> 1 350 6.67e 0
#> 2 435 4.32e 1
#> 3 4800 1.94e43
#> 4 515 2.50e 2